From Blogsphere to a Static Site (Part 1) - Objects
The migration tooling revolves around data, so getting the data classes right is important. The data classes need to be designed to allow it to be populated either from the Blogsphere NSF or from a collection of JSON files (so the blog generation can continue when the NSF is gone). For the blog we need 3 objects:
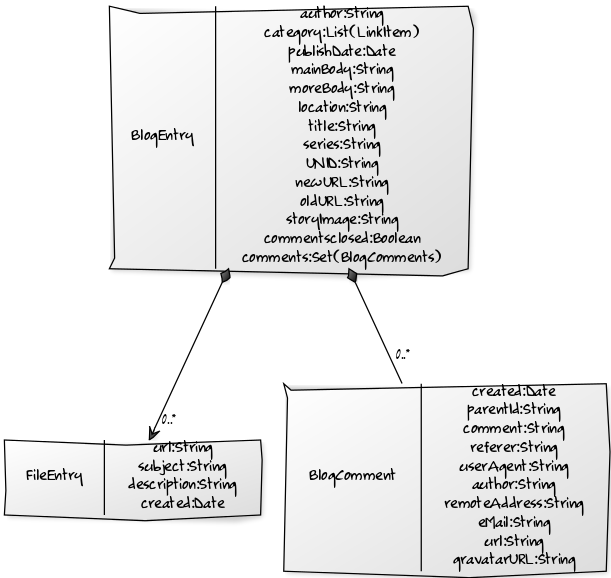
The interesting part is the call to the
- BlogEntry: The main data containing a blog entry including its meta data
- BlogComment: An entry with a comment for a Blog in a 1:n relation
- FileEntry: information about downloadable files (needed for export)
There will be auxiliary data classes like Config, RenderInstructions, Blogindex. Their content is derived from the data stored in the main object or, in case of Config, from disk.
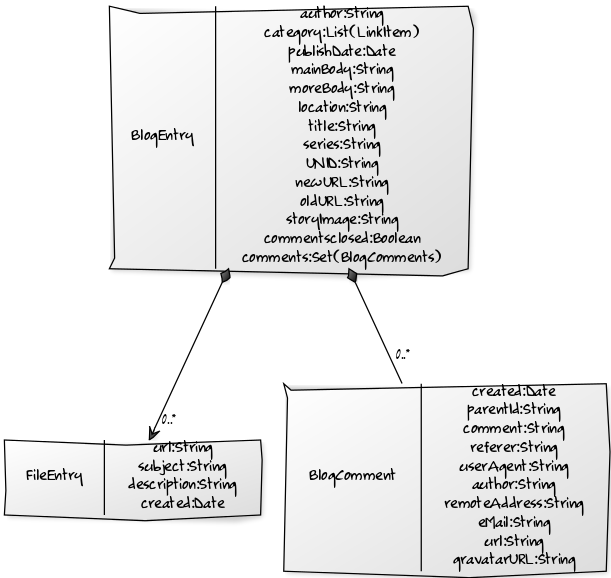
The classes all feature static methods to load from a stream and save them as JSON to a stream. I found this pattern quite efficient.
Saving as JSON
/**
* Save the object to a JSON file for reuse
*/
public void saveDatatoJson(OutputStream out) {
GsonBuilder gb = new GsonBuilder();
gb.setPrettyPrinting();
gb.disableHtmlEscaping();
Gson gson = gb.create();
PrintWriter writer = new PrintWriter(out);
gson.toJson(this, writer);
writer.flush();
writer.close();
}
@Override
public String toString() {
ByteArrayOutputStream out = new ByteArrayOutputStream();
this.saveDatatoJson(out);
return out.toString();
}
/**
* Save the object to a JSON file for reuse
*/
public void saveDatatoJson(OutputStream out) {
GsonBuilder gb = new GsonBuilder();
gb.setPrettyPrinting();
gb.disableHtmlEscaping();
Gson gson = gb.create();
PrintWriter writer = new PrintWriter(out);
gson.toJson(this, writer);
writer.flush();
writer.close();
}
@Override
public String toString() {
ByteArrayOutputStream out = new ByteArrayOutputStream();
this.saveDatatoJson(out);
return out.toString();
}
Loading from JSON
public static BlogEntry loadDataFromJson(InputStream in) {
BlogEntry result = null;
Gson gson = new GsonBuilder().create();
result = (BlogEntry) gson.fromJson(new InputStreamReader(in), BlogEntry.class);
// The comments might not be sorted on disk, so we sort them out here
Set<BlogComments> bc = new TreeSet<BlogComments>();
bc.addAll(result.getComments());
result.setComments(bc);
return result;
}
The interesting part is the call to the
fromJson()
method that reads and converts the JSON to the object defined by the class in the parameter. Gson will ignore unknown fields, so the mechanism is quite robust. Still you want to wrap that into an appropriate error handler. Next stop: collections needed for renderingPosted by Stephan H Wissel on 15 April 2017 | Comments (0) | categories: Blog